To send email in Android we can use
Intent.ACTION_SENDTO to call existing email app.
Have a look at below code snippet for Implementation
Source Code:
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="16dp"
tools:context=".MainActivity">
<TextView
android:id="@+id/tvTo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:text="@string/to"/>
<EditText
android:id="@+id/edtTo"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/tvTo"
android:inputType="textEmailAddress"
android:ems="10"/>
<TextView
android:id="@+id/tvSubject"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_below="@+id/edtTo"
android:text="@string/subject"/>
<EditText
android:id="@+id/edtSubject"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/tvSubject"/>
<TextView
android:id="@+id/tvComposeEmail"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:layout_below="@id/edtSubject"
android:text="@string/compose_email"/>
<EditText
android:id="@+id/edtComposeEmail"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/tvComposeEmail"
android:gravity="bottom"
android:lines="5" />
</RelativeLayout>
MainActivity.java
package com.sonevalley.sushil.sendingemail;
import android.content.Intent;
import android.net.Uri;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
EditText edtTo;
EditText edtSubject;
EditText edtComposeEmail;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
edtTo = (EditText) findViewById(R.id.edtTo);
edtSubject = (EditText) findViewById(R.id.edtSubject);
edtComposeEmail = (EditText) findViewById(R.id.edtComposeEmail);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int id = item.getItemId();
if (id == R.id.action_send) {
composeEmail();
}
return super.onOptionsItemSelected(item);
}
public void composeEmail() {
String[] addresses = {edtTo.getText().toString()};
String subject = edtSubject.getText().toString();
String emailCompose = edtComposeEmail.getText().toString();
Intent intent = new Intent(Intent.ACTION_SENDTO);
intent.setData(Uri.parse("mailto:")); // only Email apps will handle this
intent.putExtra(Intent.EXTRA_EMAIL, addresses);
intent.putExtra(Intent.EXTRA_SUBJECT, subject);
intent.putExtra(Intent.EXTRA_TEXT, emailCompose);
if (intent.resolveActivity(getPackageManager()) != null) {
startActivity(intent);
}
}
}
Screen Preview:
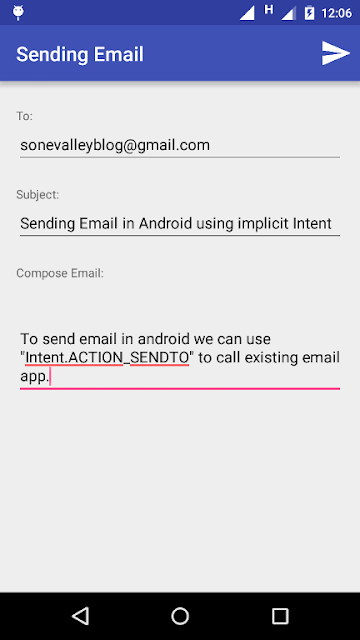 |
Sending Email in Android using Implicit Intent |
 |
Open existing email app to send email |
0 comments:
Post a Comment